I wrote a bash script for my son to convert his HEIC images to PNG so I decided to ask ChatGPT AI to do it in PowerShell for the hell of it. Well… I asked ChatGPT this question: “write a powershell script that will convert heic image files to png”
Looks like it’s time to retire! Right after I write a PowerShell module for ImageMagick as I don’t think one exists. So you’ll likely have to execute ImageMagic commands like you would in the terminal but this should work if you switch out the module calls with “& convert-image“. I don’t actually know as I don’t have a Windows machine to test with and I am not installing PowerShell on my Linux machines to find out. I should had regenerated it to see if it got it right. I bet it would.
Here’s a screenshot.
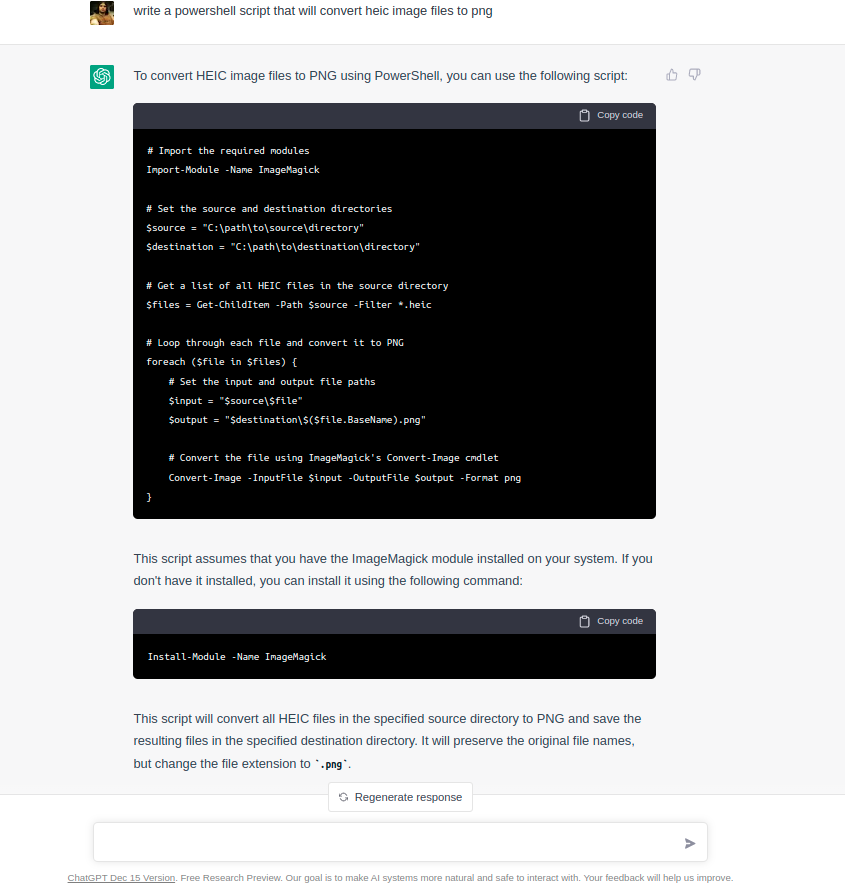
ChatGPT Textual Result
To convert HEIC image files to PNG using PowerShell, you can use the following script:
# Import the required modules Import-Module -Name ImageMagick # Set the source and destination directories $source = "C:\path\to\source\directory" $destination = "C:\path\to\destination\directory" # Get a list of all HEIC files in the source directory $files = Get-ChildItem -Path $source -Filter *.heic # Loop through each file and convert it to PNG foreach ($file in $files) { # Set the input and output file paths $input = "$source\$file" $output = "$destination\$($file.BaseName).png" # Convert the file using ImageMagick's Convert-Image cmdlet Convert-Image -InputFile $input -OutputFile $output -Format png }
This script assumes that you have the ImageMagick module installed on your system. If you don’t have it installed, you can install it using the following command:
Install-Module -Name ImageMagick
This script will convert all HEIC files in the specified source directory to PNG and save the resulting files in the specified destination directory. It will preserve the original file names, but change the file extension to .png
.